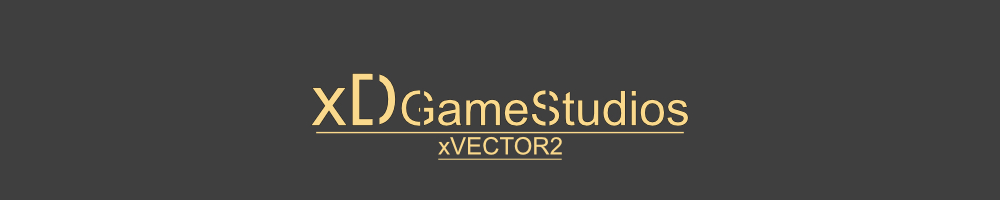
xVector2
A downloadable asset pack
[INFORMATION]
This is a Vector2 Class asset libary with a good amount of functionality to get you covered for most tasks (GMS 2.3+)
[QUICK GUIDE]
1) This module implements a constructor class so you just need to do:
var _vector = new Vector2(10, 10); // Creates a x: 10, y: 10 vector var _newVector = _vector.multiplyBy(2); // Multiplies "vector" by 2 // NOTE: methods don't mutate the current instance. // Meaning a new vector is created everytime, keeping the original vector unchanged.
2) However you may want to change the original vector. For your convenience I've included a Vector2Utils class:
var _vector = new Vector2(3, 7); Vector2Utils.normalize(_vector); // mutates the original vector. // NOTE: This allows every-step computations to keep a lighter impact on the GC.
[MANUAL]
This library provides a set of useful function that you can use to create and manipulate Vector2:
var _vector = new Vector2(10, 10); // returns the length of the vector _vector.length() // returns the squared length of the vector _vector.legnthSquared() // returns a new vector with the given magnitude. _vector.withMagnitude(magnitude) // return a new normalized vector. _vector.normalize() // returns a new reflected vector according to a normal vector. _vector.reflect(normal) // returns a new vector with clamped x/y components. _vector.limit(min, max) // return a new vector "lerped" towards the dest by a given amount. _vector.interpolate(dest, amount) // returns a new vector with the sum result. _vector.add(vector); // returns a new vector with the subtraction result. _vector.subtract(vector) // returns a new vector with the multiplication result. _vector.multiply(vector) // returns a new vector multiplied by a single value. _vector.multiplyBy(amount) // returns a new vector with the division result. _vector.divide(vector) // returns a new vector divided by a single value. _vector.divideBy(amount) // returns a new negated vector (-x, -y). _vector.negate(); // equality check between two vector2 instances. _vector.equals(vector) // stores the vector elements in a given array starting at the given index. _vector.copyToArray(array, index)
Regarding the mutable functions inside the Vector2Utils namespace the functionality is very much the same. Please check the fully documented source code.
[COMPATIBILITY]
The asset is fully compatible with GMS2.3+ and is purely written in GML making it compatible with all exports available.
Download
Click download now to get access to the following files:
Leave a comment
Log in with itch.io to leave a comment.